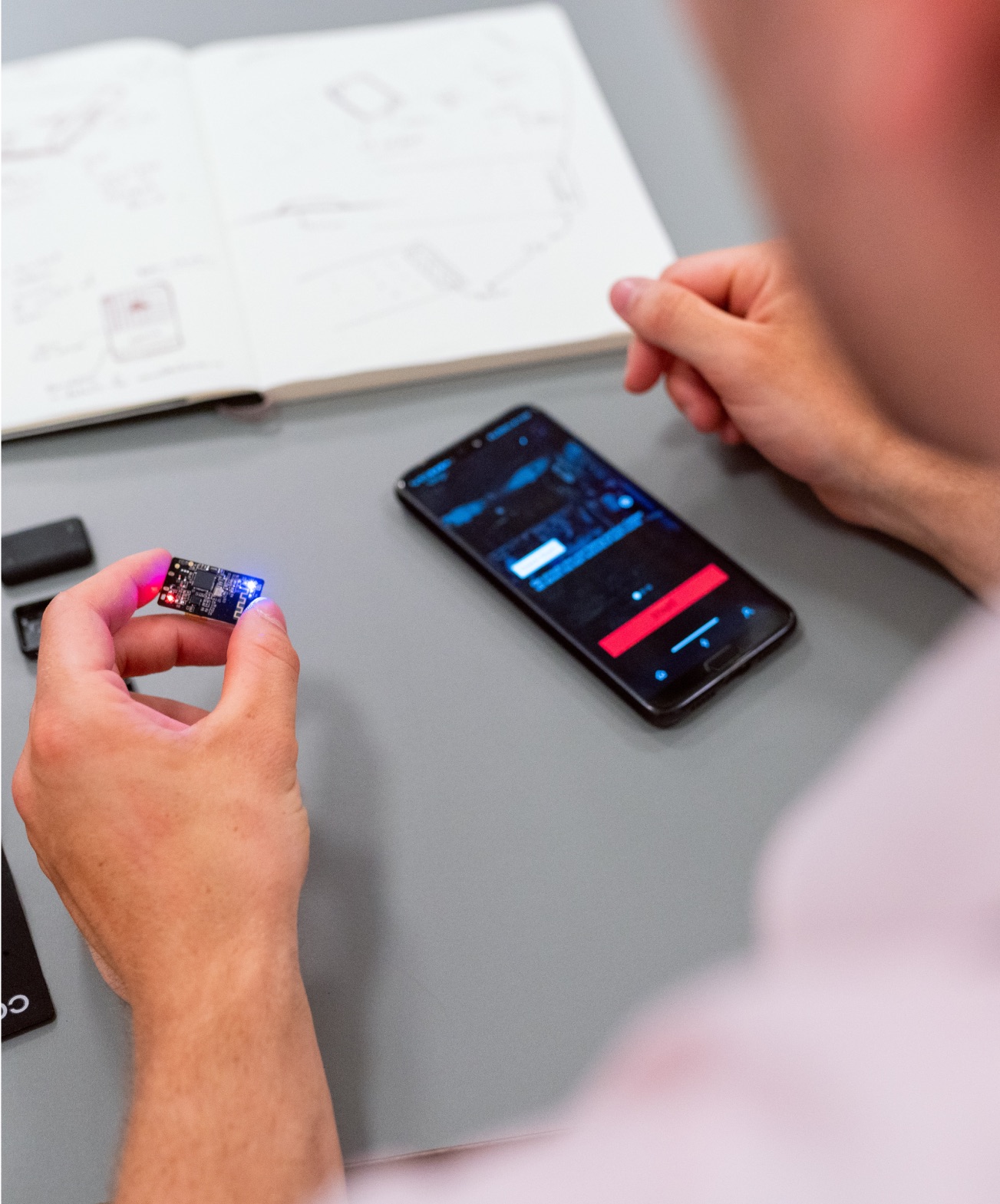
The Course
Dive right into the world of server-side development with this exciting journey tailored for newcomers. With hands-on examples, you'll learn the nuts and bolts of Node.js, a powerful JavaScript runtime beloved for its efficiency and scalability. From setting up your development environment to mastering asynchronous programming with callbacks and promises, we've got you covered. We'll explore essential modules, express web frameworks, and how to interact with databases to build dynamic web applications. Plus, you'll even get your feet wet with building simple APIs, all while writing clean, maintainable code.
Understanding Node.js not only opens up doors to back-end development but also arms you with the tools to create full-stack applications. By the end of this course, you'll have the confidence to tackle real-world projects, whether you're jazzing up your personal portfolio or stepping into the job market. Embrace the versatility of Node.js and watch as it transforms your coding skills, enabling you to build high-performance applications that can handle the demands of today's interactive websites and services. Jump into this adventure, and let's turn those lines of code into building blocks for something amazing!
What you will learn
When I crafted this course, I was keen on making the world of server-side development approachable to newcomers like yourself. Whether you're dreaming of crafting your own web applications or simply keen to add a robust skill to your toolkit, this journey you're about to embark on is meticulously organized with those thoughts in mind. The modules progress in a way where each new concept builds on the previous one, so you're never thrown into the deep end. I've handpicked practical examples and exercises that not only help you grasp the fundamentals but also allow you to see your progress in real-time. With the knowledge you'll gain, you'll be well-equipped to join the ranks of developers who are making waves with Node.js, and I'm here to guide you every step of the way. So, let's get started; I can't wait to see all the amazing things you'll build!
Curriculum
- 38. Database Intro (5:28)
- 39. Installing MongoDB in Windows (9:08)
- 40. Installing MongoDB in a Mac (8:01)
- 41. Connecting to MongoDB using the MongoDB Client (5:09)
- 42. Connecting to MongoDB using an ODM - Mongoose (8:23)
- 43. Let's learn some Database Vocabulary (8:02)
- 44. MongoDB Client - Inserting Data (6:53)
- 45. The ObjectID (9:35)
- 46. MongoDB Client - Fetching (1:21)
- 47. MongoDB Client - Updating (10:02)
- 48. MongoDB Client - Deleting (8:18)
- 49. PostMan Installation (3:58)
- 50. Mongoose - ODM - Setup (4:39)
- 51. Mongoose - creating a Model part 1 (10:32)
- 52. Mongoose - creating a Model part 2 - Data Insertion and Validation (6:56)
- 53. Mongoose - creating a Model part 3 - Using the Schema Object (5:27)
- 54. Setting up Express (4:20)
- 55. Creating Routes part 1 (3:28)
- 56. Creating Routes part 2 - saving raw data (8:18)
- 57. Setting up our code to save POST data (5:40)
- 58. Saving POST data (8:03)
- 59. Fetching Data (4:17)
- 60. Updating Data with PATCH (13:15)
- 61. Updating Data with PUT (8:33)
- 62. Deleting Data (10:34)
- 63. Intro and Modules Downloads (4:29)
- 64. Setting up Express Server and our Database (5:07)
- 65. Creating Our User Model (5:13)
- 66. Registration part 1 - Creating our register route (3:49)
- 67. Registration part 2 - Adding our Body Parser (4:31)
- 68. Registration part 3 - Saving the User (3:32)
- 69. Registration part 4 - Let's Hash the Password (7:27)
- 70. Login part 1 - Setting up the Login route (5:38)
- 71. Login part 2 - Testing User Login (3:32)
- 72. Project directories setup (6:34)
- 73. Initializing our server (5:50)
- 74. Setting up Home page (17:46)
- 75. Dynamic page content and getting styles to work (9:17)
- 76. Including Partials (4:37)
- 77. Creating our Login and Registration Views (4:34)
- 78. Build home page views (8:10)
- 79. Admin Setup part 1 - Loading and using routes (12:11)
- 80. Admin Setup part 2 - Default layout (6:03)
- 81. Admin Setup part 3 - partials and links (9:58)
- 82. Admin Setup part 4 - Javascript files (6:32)
- 83. Creating Post routes part 1 (6:04)
- 84. Let's create a database connection (9:55)
- 85. Creating the form part 1 (8:29)
- 86. Creating the form part 2 (7:36)
- 87. Creating a Post Model part 1 (9:11)
- 88. Creating a Post Model part 2 (4:14)
- 89. Including body-parser and testing (6:34)
- 90. Testing our Post Model (6:02)
- 91. Saving a Post (9:34)
- 92. Reading data part 1 - Form creation (4:20)
- 93. Reading Data part 2 - Reading Data (5:18)
- 94. Updating part 1 (6:26)
- 95. Updating part 2 - Displaying data back to the form (10:58)
- 96. Updating part 3 - Handlebars function setup (7:22)
- 97. Updating part 4 - Handlebars function finished (9:05)
- 98. Updating part 5 - Method Override Module (7:06)
- 99. Updating part 6 - Finally updating :) (6:40)
- 100. Deleting Posts (5:09)
- 101. Dummy Data creation part 1 - setup (10:06)
- 102. Dummy Data creation part 2 - Finished (14:22)
- 103. Installing and setting up the Upload Module (3:29)
- 104. Testing the FILES Object (5:17)
- 105. Uploading a file (5:47)
- 106. Creating a helper function to test Empty objects (9:31)
- 107. Inserting the file reference to the database (5:55)
- 108. Modifying duplicate pictures to have different names (4:07)
- 109. Displaying the uploaded pictures (3:21)
- 110. Deleting the files / images with the post (7:32)
- 127. Views routes and setup (10:43)
- 128. Categories Index - Create Form (4:50)
- 129. Categories Index - Display Form (4:45)
- 130. Creating a category (4:10)
- 131. Displaying categories (2:49)
- 132. Categories edit part 1 - Link (4:13)
- 133. Categories edit part 2 - Edit View (4:13)
- 134. Categories - Updating (5:02)
- 135. Categories - Deleting (3:01)
- 136. Displaying Categories in Home Page (5:34)
- 137. Adding a Select to the Edit Post View (3:43)
- 138. Adding a Select to the Create Post View (2:58)
- 139. Finishing up with Categories (9:14)
- 140. Auth Intro (2:58)
- 141. Creating our User Model (4:24)
- 142. Adding our User to the Post Route (5:28)
- 143. Adding some Validation (11:36)
- 144. Registering a User (3:43)
- 145. Hashing User's password with a module - part 1 (8:47)
- 146. Hashing User's password with a module - part 2 (3:32)
- 147. Adding Flash notification for registration (2:31)
- 148. User already exists feature (10:09)
- 149. Moving Database Config and Post login route (4:32)
- 150. Passport Module part 1 - Login route setup (5:27)
- 151. Passport Module part 2 - Testing (6:14)
- 152. Passport Module part 3 - Verifying users (8:59)
- 153. Passport Module part 3 - Login users in (8:28)
- 154. Displaying Logged-In User and Errors (6:09)
- 155. Login out (2:37)
- 156. Login out Modal (2:29)
- 157. Protecting our Admin Routes (5:48)
- 158. Model relationships (5:44)
- 159. Create route part 1 - form (6:00)
- 160. Create route part 2 - creating comments (13:31)
- 161. Setting up our Comment index (5:46)
- 162. Displaying Comments (5:35)
- 163. Displaying Comment Owner and formatting date (4:17)
- 164. Deleting comments (5:46)
- 165. Deleting Post with comments (6:34)
- 166. Displaying only logged in user comments (2:14)
- 167. Deleting comment references in the Post documents (7:23)
- 168. Hiding comments if not allowed (7:33)
- 169 Displaying comments front end (6:54)
- 170. Populating users in comments (5:49)
- 171. Populating user for posts (6:58)
- 172. Creating a user specific page for Posts (11:18)
- 173. Installing Bootstrap Buttons Plugin (6:12)
- 174. Initializing our Button (4:30)
- 175. Listening for the Change Event (4:40)
- 176. Sending the AJAX request - Test (7:56)
- 177. Getting Data (6:15)
- 178. Finally Updating with AJAX (2:37)
- 179. Adding some Notifications with this AWESOME JS Library (5:13)
- 180. Displaying only approved comments (5:07)
- 181. Adding Flash notification to comments (3:06)
- 187. Creating handlebars helper function and testing (7:00)
- 188. Route modification (5:06)
- 189. Creating dynamic list items in Handlebars function part 1 (5:58)
- 190. Creating dynamic list items in Handlebars function part 2 (6:55)
- 191. Creating dynamic list items in Handlebars function part 3 (5:37)
- 192. Creating dynamic list items in Handlebars function part 4 (3:56)
Your instructor
As the spearhead of the "Node.js for Beginners - Become a Node.js Developer" course, I, Coding Admin, bring a robust background in JavaScript and server-side development to the classroom. With years of real-world experience harnessing the power of Node.js in creating scalable and efficient web applications, my journey has been marked by a passion for educating and empowering upcoming developers. Through hands-on projects and pivotal roles in tech startups and established firms, I've developed a deep-seated understanding of Node.js that I am eager to pass onto my students.
My connection to this course runs deep, as it epitomizes the very environment where my expertise and my educational aspirations converge. I am committed to breaking down complex concepts into digestible, engaging lessons that catalyze the growth of aspiring developers. By sharing the challenges I've overcome and the insights gained from working with Node.js, I aim to foster a learning community that's as excited about real-time web development as I am. Through each module of this course, I will guide students to build a solid foundation, ultimately equipping them with the skills needed to pave their own path as Node.js developers.

Comprehensive
Mastering Node.js: From Fundamentals to Advanced Concepts
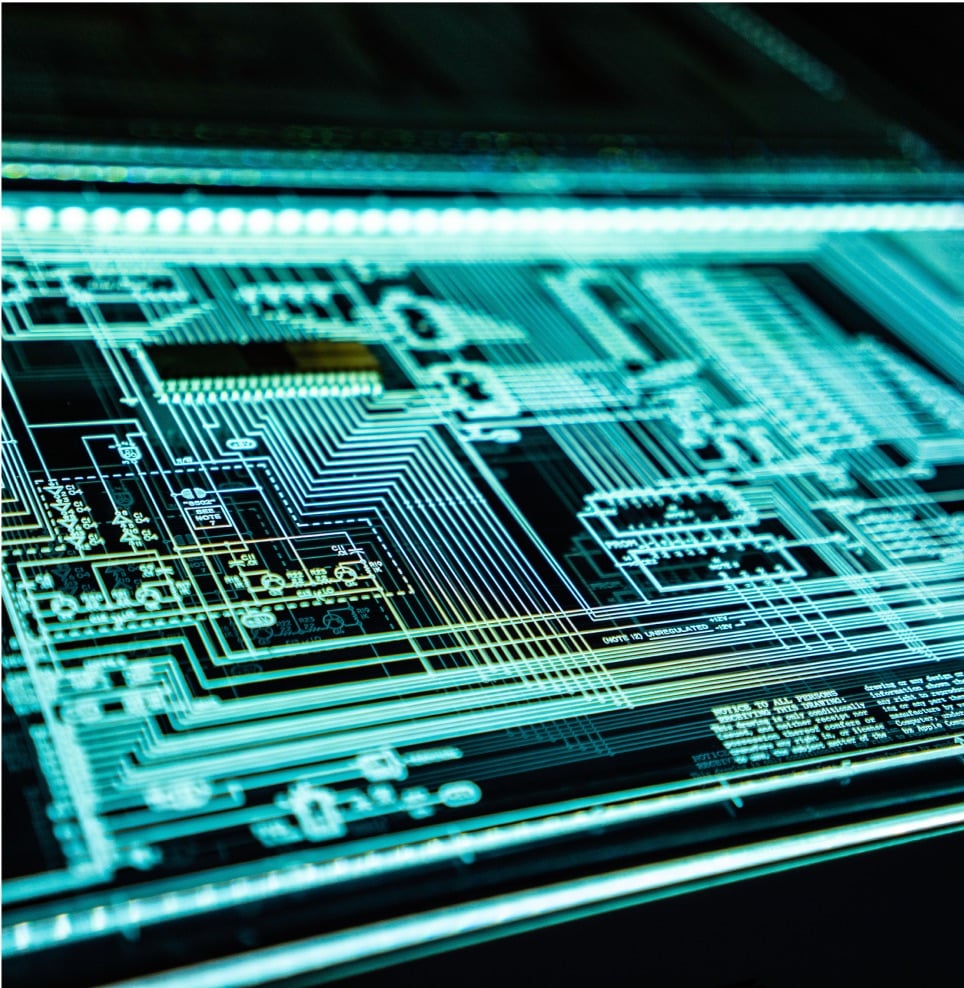
Hands-On
Node.js Essentials: Build Real-World Applications
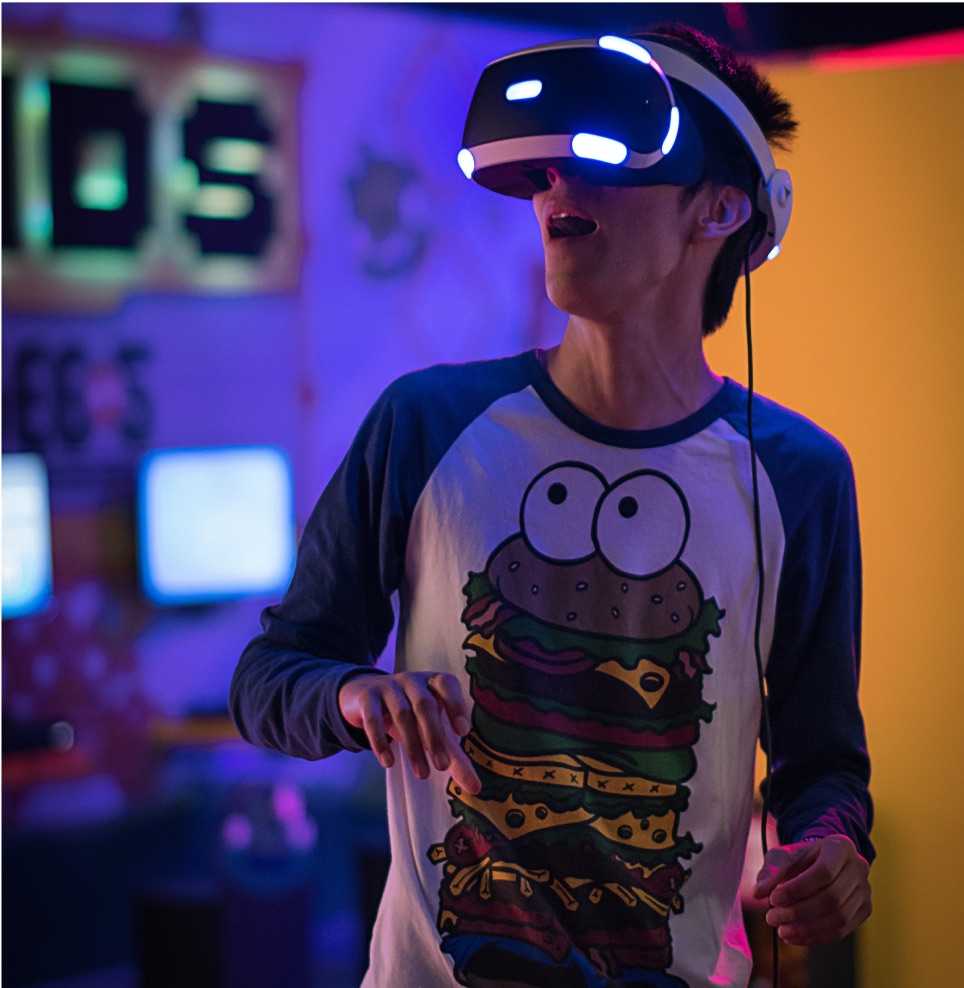
Streamlined
Node.js Fundamentals: Quickstart Development Guide